OCI CLI Authentication
- Session Token Based
- You must use the
--auth security_token
or set theOCI_CLI_AUTH
environment variable tosecurity_token
to authenticate CLI commands using the session token. - To validate, run ==> oci session validate –config-file ${file location} –profile ${profile name} –auth security_token
- You must use the
- API key based (less secure)
OCI CLI and Configure
on mac
brew install oci
After installation, run configuration command. This will collect your ocids for your user, tenancy , region and more. when all done, it will create 3 file in .oci folder. 1 config file and two .pem files.
oci setup config
.
.
.
Enter a location for your config [/Users/work/.oci/config]:
Enter a user OCID:
Enter a tenancy OCID:
Enter a region by index or name:
.
.
.
# at the end you will have two a .pem files created on your system
# like this
cd /Users/${Your username}/.oci
ls -l
#output
-rw------- 1 work staff 316 Jan 29 13:34 config
-rw------- 1 work staff 1704 Jan 29 13:34 oci_chaoyuim001_api_key.pem
-rw------- 1 work staff 451 Jan 29 13:34 oci_chaoyuim001_api_key_public.pem
The oci_chaoyuim001_api_key_public.pem file will be used to upload to OCI console user.
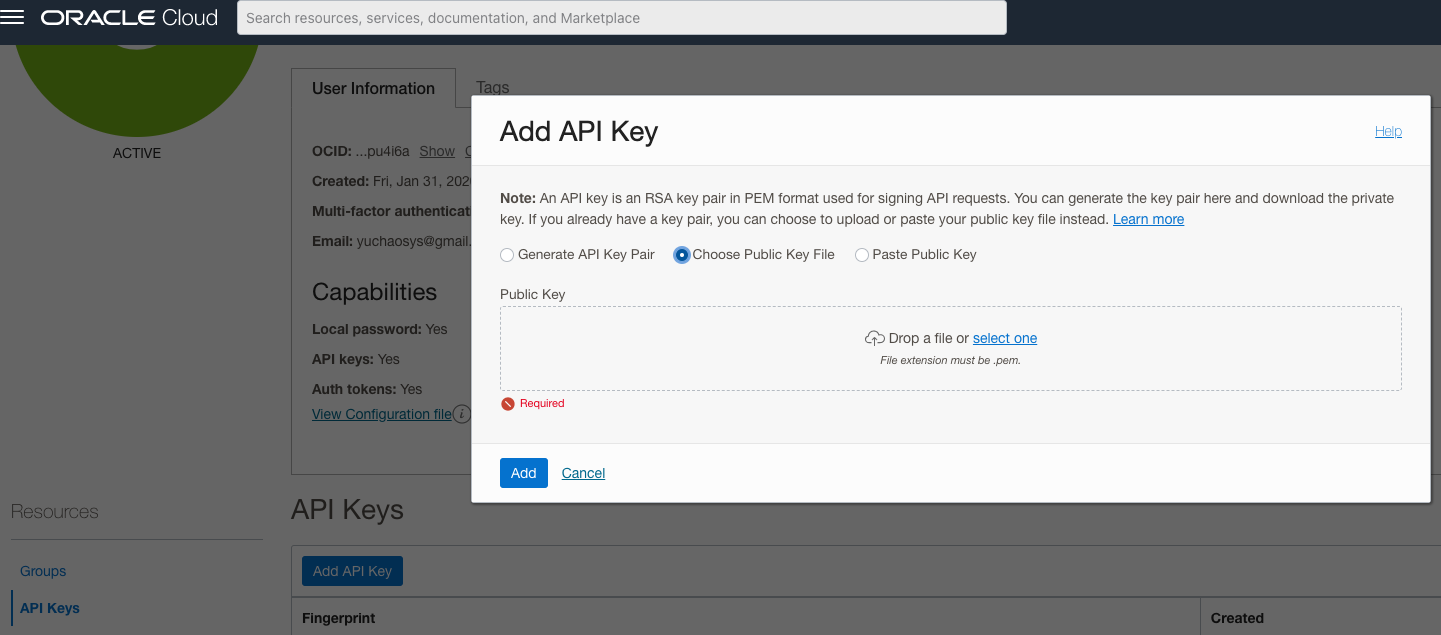
Click on it to validate your local config file ( cat /Users/${Your username}/.oci/config )
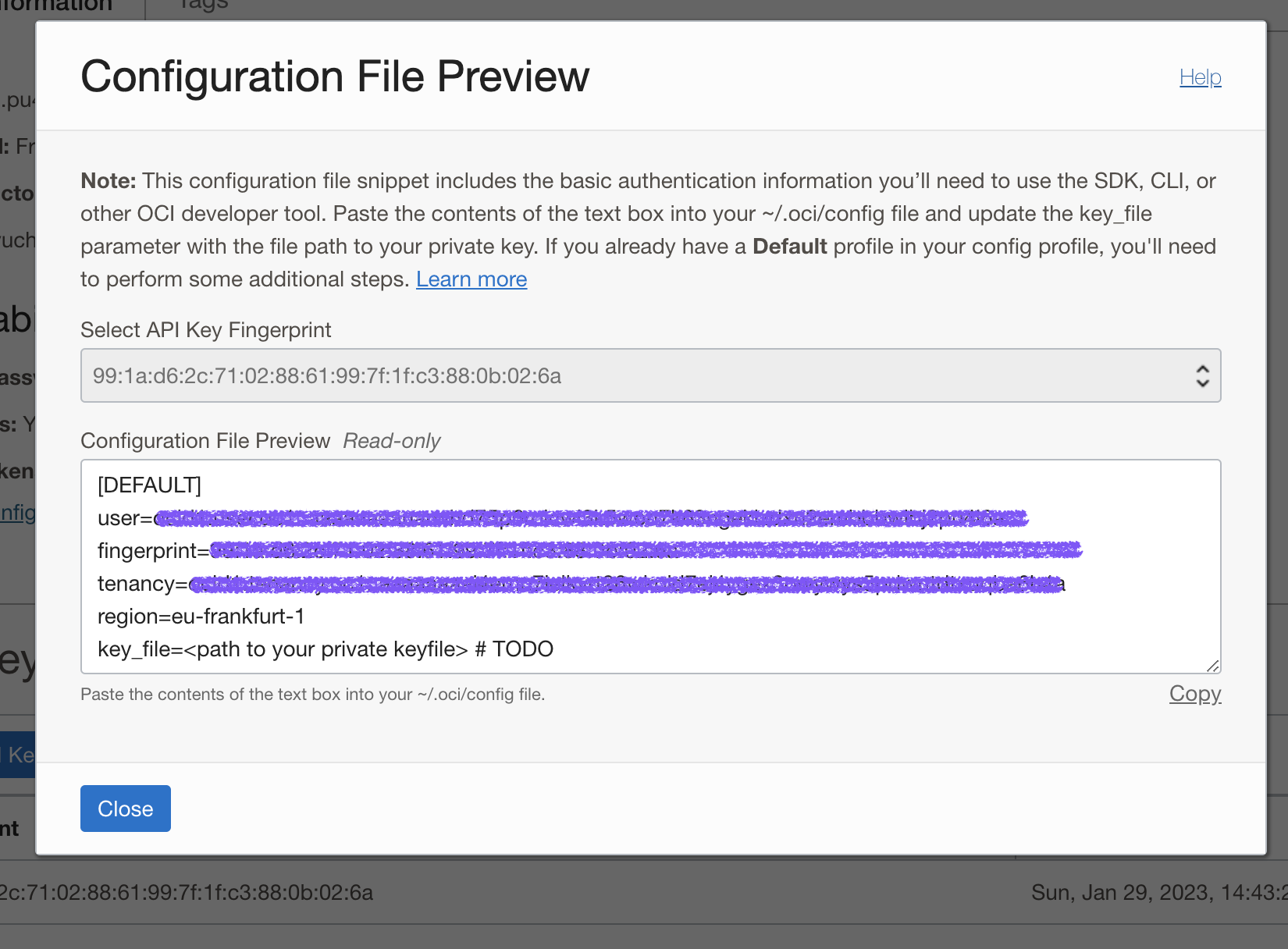
A Quick Exmple of Displaying all buckets in Compartment
oci os bucket list --all -c ${your compartment ocid} --query 'data[*].[name,namespace]' --output table
+----------------------+--------------+
| Column1 | Column2 |
+----------------------+--------------+
| bucket-20230129-1938 | frpnibrn7ulj |
| test | frpnibrn7ulj |
+----------------------+--------------+
Example Create buckets with autotiering enabled
oci os bucket create -c ${your compartment ocid} --auto-tiering InfrequentAccess --name test22
How Start / Stop VMs and Autonomous Databases in OCI with OCI CLI
.sh to stop and start VMs once attached to a schedule cron job, this can act as agent to manage your resources.
#!/bin/bash
Help() {
# Display Help
echo "this .sh file takes 3 parameters \$compartment-id \$action_type \$skip"
echo "\$compartment-id \$action_type are mandatory"
echo "\$skip can be used if the start / stop action should skip one or more oci resources"
echo "\$compartment-id is the ocid of a compartment"
echo "\$action_type can be either START or STOP"
echo "\$skip is the display-name of oci resouces, more than 1 can be supplied and seperated by "," "
echo -e '\n'
echo -e 'example \nbash vm_auto_start_or_stop.sh ${compartment_id} START vm001,vm002,v003'
echo 'this will try to start any vms NOT named as "vm001,vm002,v003" if they are in stopped state.'
echo -e '\n'
}
while getopts ":h" option; do
case $option in
h) # display Help
Help
exit
;;
esac
done
#############################################
COMPARTMENT_ID="$1"
ACTION_TYPE="$2"
SKIP_VM_NAMES="$3"
TEMP_FILE_NAME='list_of_instance_status'
STATUS_RUNNING='RUNNING'
STATUS_STOPPED='STOPPED'
LOG_FILE_NAME='vms_log.txt'
echo " $(date +'%m/%d/%Y %T') ############## compartment_id => $COMPARTMENT_ID action_type => $ACTION_TYPE skip => $SKIP_VM_NAMES ####################" >>$LOG_FILE_NAME
if [ -z "$COMPARTMENT_ID" ] | [ -z "$ACTION_TYPE" ]; then
echo "\$COMPARTMENT_ID must NOT be empty"
echo "\$ACTION TYPE must NOT be empty"
echo -e "run: \nbash vm_auto_start_or_stop.sh -h\nfor help"
exit 1
fi
if [ "$ACTION_TYPE" != "START" ] && [ "$ACTION_TYPE" != "STOP" ]; then
echo -e "ACTION_TYPE can only be START or STOP"
exit 1
fi
oci compute instance list \
--compartment-id $COMPARTMENT_ID \
--query 'data[*].["display-name","lifecycle-state","id"]' \
--output table >$TEMP_FILE_NAME
cat $TEMP_FILE_NAME | while read line; do
INSTANCE=$(echo $line | cut -d'|' -f 4 | xargs)
INSTANCE_NAME=$(echo $line | cut -d'|' -f 2 | xargs)
if [[ "$line" == *"$STATUS_RUNNING"* || "$line" == *"$STATUS_STOPPED"* ]]; then
if [[ "$SKIP_VM_NAMES" == *"$INSTANCE_NAME"* ]]; then
echo -e "\n"
echo "${INSTANCE_NAME} is FOUND in '$SKIP_VM_NAMES'"
echo "DO nothing with ${INSTANCE_NAME}"
else
echo -e "\n"
echo "${INSTANCE_NAME} is NOT FOUND in '$SKIP_VM_NAMES'"
echo "START or STOP ${INSTANCE_NAME}"
if [[ "$line" == *"$STATUS_RUNNING"* && "$ACTION_TYPE" = "STOP" ]]; then
echo "running instance found"
sleep 1
echo "issue STOP action to this instance ==> $INSTANCE_NAME"
sleep 5
oci compute instance action \
--action STOP \
--instance-id $INSTANCE \
--query 'data.["display-name","lifecycle-state","id"]' \
--output table >>$LOG_FILE_NAME
sleep 5
echo "."
echo ".."
sleep 1
echo "."
echo ".."
elif [[ "$line" == *"$STATUS_STOPPED"* && "$ACTION_TYPE" = "START" ]]; then
echo "Stopped instance found"
sleep 1
echo "issue start action to this instance ==> $INSTANCE_NAME"
sleep 5
oci compute instance action \
--action START \
--instance-id $INSTANCE \
--query 'data.["display-name","lifecycle-state","id"]' \
--output table >>$LOG_FILE_NAME
sleep 5
echo "."
echo ".."
sleep 1
echo "."
echo ".."
fi
fi
fi
done
#!/bin/bash
Help() {
# Display Help
echo "this .sh file takes 3 parameters \$compartment-id \$action_type \$skip"
echo "\$compartment-id \$action_type are mandatory"
echo "\$skip can be used if thie start / stop action should skip one or more oci resources"
echo "\$compartment-id is the ocid of a compartment"
echo "\$action_type can be either START or STOP"
echo "\$skip is the display-name of oci resouces, more than 1 can be supplied and seperated by "," "
echo -e '\n'
echo -e 'example \nbash vm_auto_start_or_stop.sh ${compartment_id} START vm001,vm002,v003'
echo 'this will try to start any vms NOT named as "vm001,vm002,v003" if they are in stopped state.'
echo -e '\n'
}
while getopts ":h" option; do
case $option in
h) # display Help
Help
exit
;;
esac
done
#############################################
COMPARTMENT_ID="$1"
ACTION_TYPE="$2"
SKIP_ATP_NAMES="$3"
TEMP_FILE_NAME='list_of_apt_status'
STATUS_AVAILABLE='AVAILABLE'
STATUS_STOPPED='STOPPED'
LOG_FILE_NAME='apt_log.txt'
echo " $(date +'%m/%d/%Y %T') ############## compartment_id => $COMPARTMENT_ID action_type => $ACTION_TYPE skip => $SKIP_ATP_NAMES ####################" >>$LOG_FILE_NAME
if [ -z "$COMPARTMENT_ID" ] | [ -z "$ACTION_TYPE" ]; then
echo "\$COMPARTMENT_ID must NOT be empty"
echo "\$ACTION TYPE must NOT be empty"
echo -e "run: \nbash vm_auto_start_or_stop.sh -h\nfor help"
exit 1
fi
if [ "$ACTION_TYPE" != "START" ] && [ "$ACTION_TYPE" != "STOP" ]; then
echo -e "ACTION_TYPE can only be START or STOP"
exit 1
fi
oci db autonomous-database list \
--compartment-id $COMPARTMENT_ID \
--query 'data[*].["display-name","lifecycle-state","id"]' \
--output table >$TEMP_FILE_NAME
cat $TEMP_FILE_NAME | while read line; do
ATP=$(echo $line | cut -d'|' -f 4 | xargs)
ATP_NAME=$(echo $line | cut -d'|' -f 2 | xargs)
if [[ "$line" == *"$STATUS_AVAILABLE"* || "$line" == *"$STATUS_STOPPED"* ]]; then
if [[ "$SKIP_ATP_NAMES" == *"$ATP_NAME"* ]]; then
echo -e "\n"
echo "${ATP_NAME} is FOUND in '$SKIP_ATP_NAMES'"
echo "DO nothing with ${ATP_NAME}"
else
echo -e "\n"
echo "${ATP_NAME} is NOT FOUND in '$SKIP_ATP_NAMES'"
echo "START or STOP ${ATP_NAME}"
if [[ "$line" == *"$STATUS_AVAILABLE"* && "$ACTION_TYPE" = "STOP" ]]; then
echo "running ATP found"
sleep 1
echo "issue STOP action to this ATP ==> $ATP_NAME"
sleep 5
oci db autonomous-database stop \
--autonomous-database-id $ATP \
--query 'data.["display-name","lifecycle-state","id"]' \
--output table >>$LOG_FILE_NAME
sleep 5
echo "."
echo ".."
sleep 1
echo "."
echo ".."
elif [[ "$line" == *"$STATUS_STOPPED"* && "$ACTION_TYPE" = "START" ]]; then
echo "Stopped ATP found"
sleep 1
echo "issue start action to this ATP ==> $ATP_NAME"
sleep 5
oci db autonomous-database start \
--autonomous-database-id $ATP \
--query 'data.["display-name","lifecycle-state","id"]' \
--output table >>$LOG_FILE_NAME
sleep 5
echo "."
echo ".."
sleep 1
echo "."
echo ".."
fi
fi
fi
done
How to copy files from One Object storage to Another
- Get a list of all the file you like to copy
- Save the list into a txt file locally
- loop through the file line by line to get the file name
- Create COPY work request for each file
- output console lines into a file to check status
# to list all objects in a bucket and output into a file
oci os object list --bucket-name MYBUCKET --all > file.txt
this policy is needed before COPY request can be created
- Allow service objectstorage-eu-amsterdam-1 to manage object-family in compartment YOURCOMPRTMENT (see bucket replication options for this policy)
echo "start";
FILE_NAME="";
I=0;
while read p; do
FILE_NAME=$(echo $p | sed 's/I//g' | sed 's/ //g');
echo $FILE_NAME;
#((I++))
#if [[ "$I" == '3' ]]
#then
# echo "Number $I";
# break;
#fi
oci os object copy --bucket-name $YOURBUCKETNAME --destination-bucket $YOURDESTBUCKETNAME --source-object-name $FILE_NAME >> copy_log.txt
done < allfiles.txt